문제 링크
주의사항
- JAVA를 사용하여 프로그램을 사용하였습니다.
- 백준에서 코드를 작성하였을 때 아래 형태에서 Main에서 결과가 출력되어야 합니다.
public class Main{
public static void main(String[] args){
}
}
문제 설명
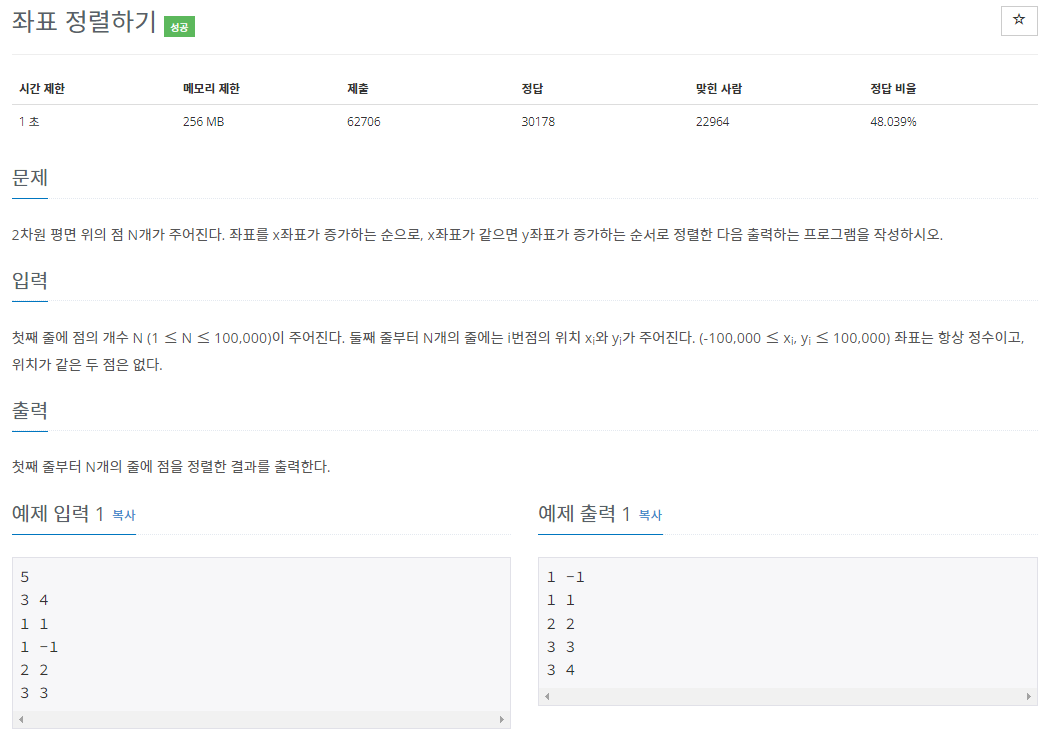
접근 방법
문제에 내용을 보고 리스트에 객체를 이용하여 Collections.sort()를 진행하면 정렬이 될 것임을 파악하였습니다.
location이라는 객체를 만들었으며 그 안에 Compartor를 만들어서 정렬하도록하였습니다.
- x좌표와 y좌표를 저장하는 객체인 location을 만들었습니다.
- 객체에 Compartor를 사용하기 위해 implementes Comparable<location>을 선언해주었습니다.
- CompareTo를 구성하였습니다.
- y좌표보다 작으면 순서를 바꾼다.
- y좌표가 같으면 x좌표를 비교하여 순서를 바꾼다.
- 이 외에는 바꾸지 않는다.
- BufferedReader를 사용하여 입력 값을 받았습니다.
- location 객체를 사용하는 ArrayList를 만들었습니다.
- StringTokenizer를 사용하여 띄어쓰기 기준으로 나누어 x좌표와 y좌표를 저장하였습니다.
- for문을 통하여 ArrayList에 값들을 저장하였습니다.
- Collections.sort()를 사용하여 리스트를 저장하였습니다.
- for문을 통해 리스트에 값을 bw에 저장하였습니다.
- BufferedWriter를 통해 저장된 결과를 출력하였습니다.
결과 코드
import java.io.*;
import java.util.*;
class location implements Comparable<location>{ //좌표 객체
int x,y;
location(int x, int y){
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
@Override
public int compareTo(location temp) { //Compartor 구성
if(this.y>temp.y)
return 1;
else if(this.y==temp.y) {
if(this.x>temp.x)
return 1;
}
return -1;
}
}
public class Main{
public static void main(String[] args) throws IOException{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
//BufferedReader를 통해 입력 값 받기
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
//BufferedWriter를 통해 결과 출력
int index = Integer.parseInt(br.readLine()); //좌표 횟수 저장
StringTokenizer st;
ArrayList<location> list = new ArrayList<location>(); //리스트 초기화
for(int i=0;i<index;i++) { //리스트에 값 저장
st = new StringTokenizer(br.readLine(), " ");
int x = Integer.parseInt(st.nextToken());
int y = Integer.parseInt(st.nextToken());
list.add(new location(x,y));
}
Collections.sort(list); //정렬
for(int i=0;i<list.size();i++) { //결과 bw에 저장
bw.write(list.get(i).getX() + " " + list.get(i).getY() + "\n");
}
bw.flush(); //결과 출력
bw.close();
br.close();
}
}
'백준' 카테고리의 다른 글
[백준] 단계별로 풀어보기(단계:12,정렬,JAVA)10814번, 나이순 정렬 (0) | 2022.01.15 |
---|---|
[백준] 단계별로 풀어보기(단계:12,정렬,JAVA)1181번, 단어 정렬 (0) | 2022.01.15 |
[백준] 단계별로 풀어보기(단계:12,정렬,JAVA)11650번, 좌표 정렬하기 (0) | 2022.01.14 |
[백준] 단계별로 풀어보기(단계:12,정렬,JAVA)1427번, 소트인사이드 (0) | 2022.01.13 |
[백준] 단계별로 풀어보기(단계:12,정렬,JAVA)2108번,통계학 (0) | 2022.01.13 |
댓글